Random
Collection of functions which deal with randomness. There are four groups:
- random number generation
- probability distributions
- sequences generators
- noise (value, gradient, simplex)
require '[fastmath.random :as r]) (
Set initial seed
31337) (r/set-seed!
0x6ed43da1 "org.apache.commons.math3.random.JDKRandomGenerator@6ed43da1"] #object[org.apache.commons.math3.random.JDKRandomGenerator
Common functions
List of the functions which work with PRNG and distribution objects:
Random number generation
irandom
,lrandom
,frandom
,drandom
irandom
, for random integer, returns longlrandom
, returns longfrandom
, for random float, returns boxed floatdrandom
, random double- First argument should be PRNG or distribution object
- With no additional arguments, functions return:
- full range for integers and longs
- \([0,1)\) for floats and doubles
- Only for PRNGs
- With one additional argument, number from \([0,max)\) range is returned
- With two additional arguments, number form \([min,max)\) range is returned
:mersenne)) ;; => -136146582
(r/irandom (r/rng :mersenne) 0 10) ;; => 7.199819724749066 (r/drandom (r/rng
For PRNGs, see more examples in PRNG > Functions section.
For Distributions, see more examples in Distributions > Functions > Examples section.
Sampling
->seq
To generate infinite lazy sequence of random values, call ->seq
on given PRNG or distribution. Second (optional) argument can limit number of returned numbers. Third (optional) argument controls sampling method (for given n
number of samples), there are the following options:
:antithetic
- random values in pairs[r1,1-r1,r2,1-r2,...]
:uniform
- spacings between numbers follow uniform distribution:systematic
- the same spacing with random starting point:stratified
- divide \([0,1]\) inton
intervals and get random value from each subinterval
take 3 (r/->seq (r/rng :mersenne))) ;; => (0.33389670116610515 0.4201635052312518 0.020276806884650833)
(:mersenne) 3) ;; => (0.1818656323152903 0.3819745118175524 0.8874413006922237) (r/->seq (r/rng
For PRNGs, see more examples in PRNG > Functions section.
For Distributions, see more examples in Distributions > Functions > Examples section.
Seed
set-seed!
,set-seed
set-seed!
mutates object if it’s supported by underlying Java implementation, can also return newly created object.set-seed
is implemented only for PRNGs currently
See examples in PRNG > Seed section.
PRNG
Related functions:
rng
,synced-rng
grandom
,brandom
irand
,lrand
,frand
,drand
,grand
,brand
->seq
set-seed
,set-seed!
randval
,flip
,flipb
,roll-a-dice
randval-rng
,flip-rng
,flipb-rng
,roll-a-dice-rng
Random number generation is based on PRNG (Pseudorandom Numeber Generator) objects which are responsible for keeping the state. All PRNGs are based on Apache Commons Math 3.6.1 algorithms.
Algorithm | Description |
---|---|
|
Mersenne Twister |
|
ISAAC, cryptographically secure PRNG |
|
WELL \(2^{512}-1\) period, variant a |
|
WELL \(2^{1024}-1\) period, variant a |
|
WELL \(2^{19937}-1\) period, variant a |
|
WELL \(2^{19937}-1\) period, variant c |
|
WELL \(2^{44497}-1\) period, variant a |
|
WELL \(2^{44497}-1\) period, variant b |
|
java.util.Random instance, thread safe |
To create PRNG, call rng
with algorithm name (as a keyword) and optional seed parameter. rng
creates a PRNG with optional seed synced-rng
wraps PRNG to ensure thread safety
:isaac) ;; => #object[org.apache.commons.math3.random.ISAACRandom 0x7e504710 "org.apache.commons.math3.random.ISAACRandom@7e504710"]
(r/rng :isaac 5336) ;; => #object[org.apache.commons.math3.random.ISAACRandom 0x5a2c5f20 "org.apache.commons.math3.random.ISAACRandom@5a2c5f20"]
(r/rng :isaac)) ;; => #object[org.apache.commons.math3.random.SynchronizedRandomGenerator 0x2e1db89c "org.apache.commons.math3.random.SynchronizedRandomGenerator@2e1db89c"] (r/synced-rng (r/rng
Functions
Two additional functions are supported by PRNGs
grandom
- 1-arity - returns random number from Normal (Gaussian) distribution, N(0,1)
- 2-arity - N(0,stddev)
- 3-arity - N(mean, stddev)
brandom
- 1-arity - returns true/false with probability=0.5
- 2-arity - returns true with given probability
All examples will use Mersenne Twister PRNG with random seed
def my-prng (r/rng :mersenne)) (
;; => -1170441606
(r/irandom my-prng) 5) ;; => 0
(r/irandom my-prng 10 20) ;; => 11
(r/irandom my-prng ;; => 7743624255826441702
(r/lrandom my-prng) 5) ;; => 1
(r/lrandom my-prng 10 20) ;; => 16
(r/lrandom my-prng ;; => 0.61668
(r/frandom my-prng) 2.5) ;; => 0.6216237
(r/frandom my-prng 10.1 10.11) ;; => 10.104496
(r/frandom my-prng ;; => 0.09798699295075952
(r/drandom my-prng) 2.5) ;; => 0.08684209039926671
(r/drandom my-prng 10.1 10.11) ;; => 10.103132178691665
(r/drandom my-prng ;; => -1.3550229774760765
(r/grandom my-prng) 20.0) ;; => 42.30684980291382
(r/grandom my-prng 10.0 20.0) ;; => -5.825627331308176
(r/grandom my-prng ;; => true
(r/brandom my-prng) 0.01) ;; => false
(r/brandom my-prng 0.99) ;; => true (r/brandom my-prng
Sampling
take 2 (r/->seq my-prng)) ;; => (0.7364928783080171 0.7459180979011766)
(2) ;; => (0.7385897820549794 0.22534289762503334)
(r/->seq my-prng 5 :antithetic) ;; => (0.7753904032017882 0.2246095967982118 0.646606217042702 0.35339378295729795 0.09059579887200875)
(r/->seq my-prng 5 :uniform) ;; => (0.2246322215775946 0.2889619056593499 0.4345685443782038 0.5766578047763047 0.5981291396129231)
(r/->seq my-prng 5 :systematic) ;; => (0.08388649375429158 0.2838864937542916 0.48388649375429155 0.6838864937542916 0.8838864937542916)
(r/->seq my-prng 5 :stratified) ;; => (0.10336014117433656 0.3872635735550631 0.4413850626051383 0.7099244429984433 0.9635487930776709) (r/->seq my-prng
Seed
Let’s define two copies of the same PRNG with the same seed. Second one is obtained by setting a seed new seed.
def isaac-prng (r/rng :isaac 9988)) (
def isaac-prng2 ;; new instance
(12345)) (r/set-seed isaac-prng
3) ;; => (0.2017025716542833 0.18388677470983206 0.956730341010493)
(r/->seq isaac-prng 3) ;; => (0.9750469483729258 0.8824640458238968 0.931330617691251)
(r/->seq isaac-prng2 3) ;; => (0.5016097251798766 0.9741775702598627 0.4876589891468033)
(r/->seq isaac-prng 3) ;; => (0.7878968013812606 0.4365686819291106 0.9175915936830081) (r/->seq isaac-prng2
Let’s now reseed both PRNGs
9988) (r/set-seed! isaac-prng
0x72ae2bf4 "org.apache.commons.math3.random.ISAACRandom@72ae2bf4"] #object[org.apache.commons.math3.random.ISAACRandom
9988) (r/set-seed! isaac-prng2
0x68f2c0f6 "org.apache.commons.math3.random.ISAACRandom@68f2c0f6"] #object[org.apache.commons.math3.random.ISAACRandom
3) ;; => (0.2017025716542833 0.18388677470983206 0.956730341010493)
(r/->seq isaac-prng 3) ;; => (0.2017025716542833 0.18388677470983206 0.956730341010493) (r/->seq isaac-prng2
Default PRNG
There is defined one global variable default-rng
which is synchonized :jvm
PRNG. Following set of functions work on this particular PRNG. The are the same as xrandom
, ie (irand)
is the same as (irandom default-rng)
.
irand
, random integer, as longlrand
, random longfrand
, random float as boxed Floatdrand
, random doublegrand
, random gaussianbrand
, random boolean->seq
, returns infinite lazy sequenceset-seed
, seeds default-rng, returns new instanceset-seed!
, seeds default-rng and Smile’s RNG
;; => 105368218
(r/irand) 5) ;; => 3
(r/irand 10 20) ;; => 19
(r/irand ;; => -6326787748595902987
(r/lrand) 5) ;; => 4
(r/lrand 10 20) ;; => 16
(r/lrand ;; => 0.20756257
(r/frand) 2.5) ;; => 0.95548046
(r/frand 10.1 10.11) ;; => 10.105913
(r/frand ;; => 0.5293197586726303
(r/drand) 2.5) ;; => 0.5856958321231587
(r/drand 10.1 10.11) ;; => 10.10447314352286
(r/drand ;; => 2.1340019834469315
(r/grand) 20.0) ;; => -33.23574732491791
(r/grand 10.0 20.0) ;; => 11.061399646894813
(r/grand ;; => false
(r/brand) 0.01) ;; => false
(r/brand 0.99) ;; => true
(r/brand take 3 (r/->seq)) ;; => (0.8820950564851541 0.8432355490117891 0.7757833765798361)
(;; => #object[org.apache.commons.math3.random.JDKRandomGenerator 0x6ed43da1 "org.apache.commons.math3.random.JDKRandomGenerator@6ed43da1"]
r/default-rng ;; => #object[org.apache.commons.math3.random.JDKRandomGenerator 0x6692a0fb "org.apache.commons.math3.random.JDKRandomGenerator@6692a0fb"]
(r/set-seed) 9988) ;; => #object[org.apache.commons.math3.random.JDKRandomGenerator 0x6a330d55 "org.apache.commons.math3.random.JDKRandomGenerator@6a330d55"]
(r/set-seed ;; => #object[org.apache.commons.math3.random.JDKRandomGenerator 0x6ed43da1 "org.apache.commons.math3.random.JDKRandomGenerator@6ed43da1"]
(r/set-seed!) 9988) ;; => #object[org.apache.commons.math3.random.JDKRandomGenerator 0x6ed43da1 "org.apache.commons.math3.random.JDKRandomGenerator@6ed43da1"] (r/set-seed!
Additionally there are some helpers:
randval
, A macro, returns value with given probability (default true/false with prob=0.5)flip
, Returns 1 with given probability (or 0)flipb
, Returns true with given probability (default probability 0.5)roll-a-dice
, Returns a result of rolling a n-sides dice(s)
;; => false
(r/randval) 0.99) ;; => true
(r/randval 0.01) ;; => false
(r/randval :value1 :value2) ;; => :value2
(r/randval 0.99 :highly-probable-value :less-probably-value) ;; => :highly-probable-value
(r/randval 0.01 :less-probably-value :highly-probable-value) ;; => :highly-probable-value
(r/randval repeatedly 10 r/flip) ;; => (0 1 1 1 0 0 1 0 0 0)
(repeatedly 10 (fn* [] (r/flip 0.8))) ;; => (1 1 1 1 1 1 1 0 1 1)
(repeatedly 10 (fn* [] (r/flip 0.2))) ;; => (0 0 0 0 0 0 0 0 0 0)
(repeatedly 10 r/flipb) ;; => (true true false true true false false true false true)
(repeatedly 10 (fn* [] (r/flipb 0.8))) ;; => (true true false true false true true false true true)
(repeatedly 10 (fn* [] (r/flipb 0.2))) ;; => (false false false false true false false false false false)
(6) ;; => 6
(r/roll-a-dice 100) ;; => 30
(r/roll-a-dice 10 6) ;; => 31 (r/roll-a-dice
Above helpers can accept custom PRNG
def isaac3-prng (r/rng :isaac 1234)) (
randval-rng
, A macro, returns value with given probability (default true/false with prob=0.5)flip-rng
, Returns 1 with given probability (or 0)flipb-rng
, Returns true with given probabilityroll-a-dice-rng
, Returns a result of rolling a n-sides dice(s)
;; => false
(r/randval-rng isaac3-prng) 0.99) ;; => true
(r/randval-rng isaac3-prng 0.01) ;; => false
(r/randval-rng isaac3-prng :value1 :value2) ;; => :value1
(r/randval-rng isaac3-prng 0.99 :highly-probable-value :less-probably-value) ;; => :highly-probable-value
(r/randval-rng isaac3-prng 0.01 :less-probably-value :highly-probable-value) ;; => :highly-probable-value
(r/randval-rng isaac3-prng repeatedly 10 (fn* [] (r/flip-rng isaac3-prng))) ;; => (0 0 0 1 1 0 0 1 0 1)
(repeatedly 10 (fn* [] (r/flip-rng isaac3-prng 0.8))) ;; => (1 0 0 0 1 1 1 1 1 1)
(repeatedly 10 (fn* [] (r/flip-rng isaac3-prng 0.2))) ;; => (1 0 0 0 1 0 0 0 0 1)
(repeatedly 10 (fn* [] (r/flipb-rng isaac3-prng))) ;; => (false false true false false true true false false true)
(repeatedly 10 (fn* [] (r/flipb-rng isaac3-prng 0.8))) ;; => (false true false true true true true true false false)
(repeatedly 10 (fn* [] (r/flipb-rng isaac3-prng 0.2))) ;; => (false false true true false false false false false false)
(6) ;; => 1
(r/roll-a-dice-rng isaac3-prng 100) ;; => 12
(r/roll-a-dice-rng isaac3-prng 10 6) ;; => 30 (r/roll-a-dice-rng isaac3-prng
Distributions
Collection of probability distributions.
Related functions:
distribution
,distribution?
,pdf
,lpdf
,observe1
cdf
,ccdf
,icdf
sample
,dimensions
,continuous?
log-likelihood
,observe
,likelihood
mean
,means
,variance
,covariance
lower-bound
,upper-bound
distribution-id
,distribution-parameters
integrate-pdf
distribution
is Distribution creator, a multimethod.
- First parameter is distribution as a
:key
. - Second parameter is a map with configuration.
:cauchy) ;; => #object[org.apache.commons.math3.distribution.CauchyDistribution 0x59c3011f "org.apache.commons.math3.distribution.CauchyDistribution@59c3011f"]
(r/distribution :cauchy {:median 2.0, :scale 2.0}) ;; => #object[org.apache.commons.math3.distribution.CauchyDistribution 0x226015a1 "org.apache.commons.math3.distribution.CauchyDistribution@226015a1"] (r/distribution
Functions
Here are the quick description of available functions:
distribution?
, checks if given object is a distributionpdf
, probability density (for continuous) or probability mass (for discrete)lpdf
, log of pdfobserve1
, log of pdf, alias of lpdfcdf
, cumulative density or probability, distributionccdf
, complementary cdf, 1-cdficdf
, inverse cdf, quantilessample
, returns random sampledimensions
, number of dimensionscontinuous?
, is distribution continuous (true) or discrete (false)?log-likelihood
, sum of lpdfs for given seq of samplesobserve
, macro version of log-likelihoodlikelihood
, exp of log-likelihoodmean
, distribution meanmeans
, distribution means for multivariate distributionsvariance
, distribution variancecovariance
, covariance for multivariate distributionslower-bound
, support lower boundupper-bound
, support upper bounddistribution-id
, name of distributiondistribution-parameters
, list of parametersintegrate-pdf
, construct cdf and icdf out of pdf function
Notes:
distribution-parameters
by default returns only obligatory parameters, when last argument istrue
, returns also optional parameters, for example:rng
drandom
,frandom
,lrandom
andirandom
work only on univariate distributionslrandom
andirandom
useround-even
to convert double to integer.cdf
,ccdf
andicdf
are defined only for univariate distributions
Examples
Let’s define Beta, Dirichlet and Bernoulli distributions as examples of univariate continuous, multivariate continuous and univariate discrete.
Log-logistic, univariate continuous
def log-logistic (r/distribution :log-logistic {:alpha 3 :beta 7})) (
;; => 15.569131250484759
(r/drandom log-logistic) ;; => 14.62328
(r/frandom log-logistic) ;; => 9
(r/lrandom log-logistic) ;; => 5
(r/irandom log-logistic) ;; => 6.775617982226123
(r/sample log-logistic) ;; => true
(r/distribution? log-logistic) 2.3) ;; => false
(r/distribution? 1) ;; => 0.008695578691184421
(r/pdf log-logistic 1) ;; => -4.744940578912748
(r/lpdf log-logistic 1) ;; => -4.744940578912748
(r/observe1 log-logistic 1) ;; => 0.0029069767441860456
(r/cdf log-logistic 1) ;; => 0.997093023255814
(r/ccdf log-logistic 0.002907) ;; => 1.0000026744341148
(r/icdf log-logistic ;; => 1
(r/dimensions log-logistic) ;; => true
(r/continuous? log-logistic) range 1 10)) ;; => -24.684504975903153
(r/log-likelihood log-logistic (range 1 10)) ;; => -24.684504975903153
(r/observe log-logistic (range 1 10)) ;; => 1.9039507073388636E-11
(r/likelihood log-logistic (;; => 8.464397033093016
(r/mean log-logistic) ;; => 46.85554132946835
(r/variance log-logistic) ;; => 0.0
(r/lower-bound log-logistic) ;; => ##Inf
(r/upper-bound log-logistic) ;; => :log-logistic
(r/distribution-id log-logistic) ;; => [{:alpha 3, :beta 7}]
(r/distribution-parameters log-logistic) true) ;; => [:rng {:alpha 3, :beta 7}] (r/distribution-parameters log-logistic
Dirichlet, multivariate continuous
def dirichlet (r/distribution :dirichlet {:alpha [1 2 3]})) (
;; => [0.003445452232110447 0.275234261853562 0.7213202859143276]
(r/sample dirichlet) reduce + (r/sample dirichlet)) ;; => 1.0
(;; => true
(r/distribution? dirichlet) 0.5 0.1 0.4]) ;; => 0.9600000000000001
(r/pdf dirichlet [0.5 0.1 0.4]) ;; => -0.040821994520255034
(r/lpdf dirichlet [0.5 0.1 0.4]) ;; => -0.040821994520255034
(r/observe1 dirichlet [;; => 3
(r/dimensions dirichlet) ;; => true
(r/continuous? dirichlet) repeatedly 10 (fn* [] (r/sample dirichlet)))) ;; => 11.705240043173438
(r/log-likelihood dirichlet (repeatedly 10 (fn* [] (r/sample dirichlet)))) ;; => 11.520175296855252
(r/observe dirichlet (repeatedly 10 (fn* [] (r/sample dirichlet)))) ;; => 600028.77038651
(r/likelihood dirichlet (;; => (0.16666666666666666 0.3333333333333333 0.5)
(r/means dirichlet) ;; => [[0.019841269841269844 -0.007936507936507936 -0.011904761904761904] [-0.007936507936507936 0.03174603174603175 -0.023809523809523808] [-0.011904761904761904 -0.023809523809523808 0.03571428571428571]]
(r/covariance dirichlet) ;; => :dirichlet
(r/distribution-id dirichlet) ;; => [:alpha]
(r/distribution-parameters dirichlet) true) ;; => [:alpha :rng] (r/distribution-parameters dirichlet
- multivariate distributions don’t implement some of the functions, like
drandom
orcdf
,icdf
, etc.
Poisson, univariate discrete
def poisson (r/distribution :poisson {:p 10})) (
;; => 8.0
(r/drandom poisson) ;; => 9.0
(r/frandom poisson) ;; => 10
(r/lrandom poisson) ;; => 11
(r/irandom poisson) ;; => 6
(r/sample poisson) ;; => true
(r/distribution? poisson) 5) ;; => 0.03783327480207072
(r/pdf poisson 5) ;; => -3.274566277811817
(r/lpdf poisson 5) ;; => -3.274566277811817
(r/observe1 poisson 5) ;; => 0.0670859628790319
(r/cdf poisson 5) ;; => 0.9329140371209681
(r/ccdf poisson 0.999) ;; => 21
(r/icdf poisson ;; => 1
(r/dimensions poisson) ;; => false
(r/continuous? poisson) range 1 10)) ;; => -35.34496599408817
(r/log-likelihood poisson (range 1 10)) ;; => -35.34496599408817
(r/observe poisson (range 1 10)) ;; => 4.46556387351644E-16
(r/likelihood poisson (;; => 10.0
(r/mean poisson) ;; => 10.0
(r/variance poisson) ;; => 0.0
(r/lower-bound poisson) ;; => 2.147483647E9
(r/upper-bound poisson) ;; => :poisson
(r/distribution-id poisson) ;; => [:p]
(r/distribution-parameters poisson) true) ;; => [:rng :p :epsilon :max-iterations] (r/distribution-parameters poisson
PDF integration
integrate-pdf
returns a pair of CDF and iCDF functions using Romberg integration and interpolation. Given interval is divided into steps
number of subinterval. Each subinteval is integrated and added to cumulative sum. All points a later interpolated to build CDF and iCDF.
Parameters:
pdf-func
- univariate function, double->doublemn
- lower bound for integration, value of pdf-func should be 0.0 at this pointmx
- upper bound for integrationsteps
- how much subintervals to integrate (default 1000)interpolator
- interpolation method between integrated points (default :linear)
Let’s compare cdf and icdf to integrated pdf of beta distribution
def beta (r/distribution :beta)) (
def integrated (r/integrate-pdf (partial r/pdf beta)
(:mn -0.001 :mx 1.001 :steps 10000
{:interpolator :spline}))
def beta-cdf (first integrated)) (
def beta-icdf (second integrated)) (
0.5) ;; => 0.890625
(r/cdf beta 0.5) ;; => 0.890624992926758
(beta-cdf 0.5) ;; => 0.26444998329511316
(r/icdf beta 0.5) ;; => 0.26444998499009115 (beta-icdf
Sampling
def weibull (r/distribution :weibull)) (
;; => 1.6077698284288813
(r/sample weibull) 3) ;; => (0.30353772759166786 0.9348549528997722 1.1235206063986871)
(r/->seq weibull 5 :antithetic) ;; => (0.8789298248121516 0.7871567720020644 0.3359031152662685 1.4959111180822753 0.5761405777290171)
(r/->seq weibull 5 :uniform) ;; => (0.27819628819981307 0.5105865483391676 0.6372189139644687 1.1425580683435526 1.7502730290537003)
(r/->seq weibull 5 :systematic) ;; => (0.42415794076771685 0.6734882729621214 0.911929539913042 1.2027888245275842 1.8282741464907257)
(r/->seq weibull 5 :stratified) ;; => (0.400168757730896 0.6539622749107047 0.891072131829612 1.1739728271224017 1.719290334861315) (r/->seq weibull
PRNG and seed
All distributions accept :rng
optional parameter which is used internally for random number generation. By default, creator constructs custom PRNG instance.
def cauchy-mersenne-2288 (r/distribution :cauchy {:rng (r/rng :mersenne 2288)})) (
vec (r/->seq cauchy-mersenne-2288 3)) ;; => [-0.1079136480774541 -5.558982159984645 -3.127061922456471]
(vec (r/->seq cauchy-mersenne-2288 3)) ;; => [8.13804818209913 1.6141542337020716 -0.6694661861436221]
(-2288 2288) ;; => #object[org.apache.commons.math3.distribution.CauchyDistribution 0x12aa7ec9 "org.apache.commons.math3.distribution.CauchyDistribution@12aa7ec9"]
(r/set-seed! cauchy-mersennevec (r/->seq cauchy-mersenne-2288 3)) ;; => [-0.1079136480774541 -5.558982159984645 -3.127061922456471] (
Default Normal
default-normal
is a public var which is a normal distribution N(0,1), thread-safe.
3) ;; => (0.08356815451083356 -1.4099400584042356 -1.5590464558923103)
(r/->seq r/default-normal 0.0) ;; => 0.3989422804014327
(r/pdf r/default-normal 0.0) ;; => 0.5
(r/cdf r/default-normal 0.5) ;; => 0.0
(r/icdf r/default-normal ;; => 0.0
(r/mean r/default-normal) ;; => 1.0 (r/variance r/default-normal)
Univariate, cont.
name | parameters |
---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Anderson-Darling
Distribution of Anderson-Darling statistic \(A^2\) on \(n\) independent uniforms \(U[0,1]\).
- Name:
:anderson-darling
- Default parameters:
:n
: \(1\)
- source
|
|||
|
Beta
|
|||
|
|||
|
|||
|
Cauchy
|
|||
|
Chi
|
|||
|
Chi-squared
|
|||
|
Chi-squared noncentral
- Name:
:chi-squared-noncentral
- Default parameters:
:nu
, degrees-of-freedom: \(1.0\):lambda
, noncentrality: \(1.0\)
- source
|
|||
|
Cramer-von Mises
Distribution of Cramer-von Mises statistic \(W^2\) on \(n\) independent uniforms \(U[0,1]\).
- Name:
:cramer-von-mises
- Default parameters
:n
: \(1\)
- source
Note: PDF is calculated using finite difference method from CDF.
|
|||
|
Erlang
|
|||
|
ex-Gaussian
- Name:
:exgaus
- Default parameters
:mu
, mean: \(5.0\):sigma
, standard deviation: \(1.0\):nu
, mean of exponential variable: \(1.0\)
- wiki, source
|
|||
|
Exponential
|
|||
|
F
- Name:
:f
- Default parameters
:numerator-degrees-of-freedom
: \(1.0\):denominator-degrees-of-freedom
: \(1.0\)
- wiki, source
|
|||
|
Fatigue life
- Name:
:fatigue-life
- Default parameters
:mu
, location: \(0.0\):beta
, scale: \(1.0\):gamma
, shape: \(1.0\)
- wiki, source
|
|||
|
Folded Normal
|
|||
|
Frechet
- Name:
:frechet
- Default parameters
:delta
, location: \(0.0\):alpha
, shape: \(1.0\):beta
, scale: \(1.0\)
- wiki, source
|
|||
|
Gamma
|
|||
|
Gumbel
|
|||
|
Half Cauchy
- Name:
:half-cauchy
- Default parameters
:scale
: \(1.0\)
- info
|
|||
|
Half Normal
- Name:
:half-normal
- Default parameters
:sigma
: \(1.0\)
- wiki
|
|||
|
Hyperbolic secant
|
|||
|
Hypoexponential
|
|||
|
Hypoexponential equal
Hypoexponential distribution, where \(\lambda_i=(n+1-i)h,\text{ for } i=1\dots k\)
- Name:
:hypoexponential-equal
- Default parameters
:k
, number of rates: \(1\):h
, difference between rates: \(1\):n
\(=\frac{\lambda_1}{h}\): \(1\)
- source
|
|||
|
Inverse Gamma
|
|||
|
Inverse Gaussian
- Name:
:inverse-gaussian
- Default parameters
|
|||
|
Johnson Sb
- Name:
:johnson-sb
- Default parameters
:gamma
, shape: \(0.0\):delta
, shape: \(1.0\):xi
, location: \(0.0\):lambda
, scale: \(1.0\)
- wiki, source
|
|||
|
Johnson Sl
- Name:
:johnson-sl
- Default parameters
:gamma
, shape: \(0.0\):delta
, shape: \(1.0\):xi
, location: \(0.0\):lambda
, scale: \(1.0\)
- source
|
|||
|
Johnson Su
- Name:
:johnson-su
- Default parameters
:gamma
, shape: \(0.0\):delta
, shape: \(1.0\):xi
, location: \(0.0\):lambda
, scale: \(1.0\)
- wiki, source
|
|||
|
Kolmogorov
![]() |
![]() |
![]() |
Kolmogorov-Smirnov
- Name:
:kolmogorov-smirnov
- Default parameters
:n
, sample size: 1
- source
|
|||
|
Kolmogorov-Smirnov+
- Name:
:kolmogorov-smirnov+
- Default parameters
:n
, sample size: 1
- source
|
|||
|
Laplace
|
|||
|
Levy
|
|||
|
Log Logistic
|
|||
|
Log Normal
|
|||
|
Logistic
|
|||
|
Nakagami
|
|||
|
Normal
|
|||
|
Normal-Inverse Gaussian
- Name:
:normal-inverse-gaussian
- Default parameters
:alpha
, tail heavyness: \(1.0\):beta
, asymmetry: \(0.0\):mu
, location: \(0.0\):delta
, scale: \(1.0\)
- wiki, source
Only PDF is supported, you may call integrate-pdf
to get CDF and iCDF pair.
|
|
![]() |
![]() |
let [[cdf icdf] (r/integrate-pdf
(partial r/pdf (r/distribution :normal-inverse-gaussian))
(:mn -800.0 :mx 800.0 :steps 5000
{:interpolator :monotone})]
0.0) (icdf 0.5)]) [(cdf
0.5000000000001334 -2.5040386431030015E-13] [
CDF | iCDF |
![]() |
![]() |
Pareto
|
|||
|
Pearson VI
|
|||
|
Power
- Name:
:power
- Default parameters
:a
: \(0.0\):b
: \(1.0\):c
: \(2.0\)
- source
|
|||
|
Rayleigh
|
|||
|
Reciprocal Sqrt
\(\operatorname{PDF}(x)=\frac{1}{\sqrt{x}}, x\in(a,(\frac{1}{2}(1+2\sqrt{a}))^2)\)
- Name:
:reciprocal-sqrt
- Default parameters
:a
, location, lower limit: \(0.5\)
|
|||
|
Student’s t
|
|||
|
Triangular
- Name:
:triangular
- Default parameters
:a
, lower limit: \(-1.0\):b
, mode: \(0.0\):c
, upper limit: \(1.0\)
- wiki, source
|
|||
|
Uniform
- Name:
:uniform-real
- Default parameters
:lower
, lower limit: \(0.0\):upper
, upper limit: \(1.0\)
- wiki, source
|
|||
|
Watson G
- Name:
:watson-g
- Default parameters
:n
: \(2\)
- source
|
|||
|
Watson U
- Name:
:watson-u
- Default parameters
:n
: \(2\)
- source
|
|||
|
Weibull
|
|||
|
Zero adjusted Gamma (zaga)
- Name:
:zaga
- Default parameters:
:mu
, location: \(0.0\):sigma
, scale: \(1.0\):nu
, density at 0.0: \(0.1\):lower-tail?
- true
- source, book
|
|||
|
Univariate, discr.
name | parameters |
---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Beta Binomial (bb)
- Name:
:bb
- Default parameters
:mu
, probability: \(0.5\):sigma
, dispersion: \(1.0\):bd
, binomial denominator: \(10\)
- wiki, source
Parameters \(\mu,\sigma\) in terms of \(\alpha, \beta\) (Wikipedia definition)
- probability: \(\mu=\frac{\alpha}{\alpha+\beta}\)
- dispersion: \(\sigma=\frac{1}{\alpha+\beta}\)
|
|||
|
Bernoulli
The same as Binomial with trials=1.
- Name:
:bernoulli
- Default parameters
:p
, probability, \(0.5\)
- wiki
|
|||
|
Binomial
|
|||
|
Fisher’s noncentral hypergeometric
- Name:
:fishers-noncentral-hypergeometric
- Default parameters
:ns
, number of sucesses: \(10\):nf
, number of failures: \(10\):n
, sample size, (\(n<ns+nf\)): \(5\):omega
, odds ratio: \(1\)
- wiki, source
|
|||
|
Geometric
|
|||
|
Hypergeometric
- Name:
:hypergeometric
- Default parameters
:population-size
: \(100\):number-of-successes
: $50%:sample-size
: $25%
- wiki, source
|
|||
|
Logarithmic
|
|||
|
Negative binomial
- Name:
:negative-binomial
- Default parameters
:r
, number of successes: \(20\), can be a real number.:p
, probability of success: \(0.5\)
- wiki
|
|||
|
|||
|
Pascal
The same as :negative-binomial
but r
is strictly integer
- Name:
:pascal
- Default parameters
:r
, number of successes: \(20\):p
, probability of success: \(0.5\)
- wiki, source
|
|||
|
Poisson
|
|||
|
Uniform
- Name:
:uniform-int
- Default parameters
:lower
, lower bound: \(0\):upper
, upper bound: \(2147483647\)
- wiki,source
|
|||
|
Zero Adjusted Beta Binomial (zabb)
- Name:
:zabb
- Default parameters
:mu
, probability: \(0.5\):sigma
, dispersion: \(0.1\):nu
, probability at 0.0: \(0.1\):bd
, binomial denominator: \(1\)
- source, book
|
|||
|
Zero Adjusted Binomial (zabi)
- Name:
:zabi
- Default parameters
:mu
, probability: \(0.5\):sigma
, probability at 0.0: \(0.1\):bd
, binomial denominator: \(1\)
- source, book
|
|||
|
Zero Adjusted Negative Binomial (zanbi)
- Name:
:zanbi
- Default parameters
:mu
, mean: \(1.0\):sigma
, dispersion: \(1.0\):nu
, probability at 0.0: \(0.3\)
- source, book
|
|||
|
Zero Inflated Beta Binomial (zibb)
- Name:
:zibb
- Default parameters
:mu
, probability: \(0.5\):sigma
, dispersion: \(0.1\):nu
, probability factor at 0.0: \(0.1\):bd
, binomial denominator: \(1\)
- source, book
|
|||
|
Zero Inflated Binomial (zibi)
- Name:
:zibi
- Default parameters
:mu
, probability: \(0.5\):sigma
, probability factor at 0.0: \(0.1\):bd
, binomial denominator: \(1\)
- source, book
|
|||
|
Zero Inflated Negative Binomial (zinbi)
- Name:
:zinbi
- Default parameters
:mu
, mean: \(1.0\):sigma
, dispersion: \(1.0\):nu
, probability factor at 0.0: \(0.3\)
- source, book
|
|||
|
Zero Inflated Poisson (zip)
|
|||
|
Zero Inflated Poisson, type 2 (zip2)
|
|||
|
Zipf
|
|||
|
Multivariate
name | parameters | continuous? |
---|---|---|
|
|
true |
|
|
true |
|
|
false |
Dirichlet
- Name:
:dirichlet
- Default parameters
:alpha
, concentration, vector:[1 1]
- wiki
Please note, PDF
doesn’t validate input.
Projections of the 2d and 3d Dirichlet distributions.
- 2d case - all vectors \([x,1-x]\)
- 3d case - all (supported) vectors \([x,y,1-x-y]\)
|
|
|
![]() |
![]() |
![]() |
|
|
|
![]() |
![]() |
![]() |
def dirichlet3 (r/distribution :dirichlet {:alpha [3 1 3]})) (
;; => [0.5003973707331635 0.11790124546181052 0.381701383805026]
(r/sample dirichlet3) ;; => [0.5675115204938257 0.11000860520108965 0.3224798743050848]
(r/sample dirichlet3) 0.2 0.3 0.5]) ;; => 1.8000000000000003
(r/pdf dirichlet3 [0.3 0.5 0.2]) ;; => 0.648
(r/pdf dirichlet3 [0.5 0.2 0.3]) ;; => 4.05
(r/pdf dirichlet3 [;; => (0.42857142857142855 0.14285714285714285 0.42857142857142855)
(r/means dirichlet3) ;; => [[0.03061224489795918 -0.007653061224489796 -0.02295918367346939] [-0.007653061224489796 0.015306122448979591 -0.007653061224489796] [-0.02295918367346939 -0.007653061224489796 0.03061224489795918]] (r/covariance dirichlet3)
Multi normal
- Name:
:multi-normal
- Default parameters
:means
, vector:[0 0]
:covariances
, vector of vectors (row-wise matrix):[[1 0] [0 1]]
- wiki, source
|
![]() |
|
![]() |
|
![]() |
Multinomial
- Name:
:multinomial
- Default parameters
:ps
, probabilities or weights, vector:[0.5 0.5]
:trials
: \(20\)
- wiki, source
def multinomial (r/distribution :multinomial {:trials 150 :ps [1 2 3 4 5]})) (
;; => [11 21 28 38 52]
(r/sample multinomial) ;; => [7 18 29 45 51]
(r/sample multinomial) 10 10 10 10 110]) ;; => 3.9088379874482466E-28
(r/pdf multinomial [10 20 30 40 50]) ;; => 8.791729390927823E-5
(r/pdf multinomial [110 10 10 10 10]) ;; => 4.955040820983416E-98
(r/pdf multinomial [;; => (10.0 20.0 30.0 40.0 50.0)
(r/means multinomial) ;; => [[9.333333333333334 -1.3333333333333333 -2.0 -2.6666666666666665 -3.333333333333333] [-1.3333333333333333 17.333333333333336 -4.0 -5.333333333333333 -6.666666666666666] [-2.0 -4.0 24.0 -8.0 -10.0] [-2.6666666666666665 -5.333333333333333 -8.0 29.333333333333336 -13.333333333333332] [-3.3333333333333335 -6.666666666666667 -10.0 -13.333333333333334 33.333333333333336]] (r/covariance multinomial)
Mixture
name | parameters |
---|---|
|
|
Mixture distribution
Creates a distribution from other distributions and weights.
- Name:
:mixture
- Default parameters:
:distrs
, list of distributions:[default-normal]
:weights
, list of weights:[1.0]
- wiki
Please note: set-seed!
doesn’t affect distributions which are part of the mixture
def three-normals
(:mixture {:distrs [(r/distribution :normal {:mu -2 :sd 2})
(r/distribution :normal)
(r/distribution :normal {:mu 2 :sd 0.5})]
(r/distribution :weights [2 1 3]}))
def mixture-of-three
(:mixture {:distrs [(r/distribution :gamma)
(r/distribution :laplace)
(r/distribution :log-logistic)]
(r/distribution :weights [2 1 3]}))
|
|||
|
;; => 0.717026180380196
(r/sample mixture-of-three) ;; => 1.4580145111592928
(r/sample mixture-of-three) 0) ;; => 0.08333333333333333
(r/pdf mixture-of-three 1) ;; => 0.45620084174033965
(r/pdf mixture-of-three 2) ;; => 0.14666525453903217
(r/pdf mixture-of-three 0) ;; => 0.08333333333333333
(r/cdf mixture-of-three 1) ;; => 0.4160780500460631
(r/cdf mixture-of-three 2) ;; => 0.6879135433937651
(r/cdf mixture-of-three 0.01) ;; => -2.1202635780176586
(r/icdf mixture-of-three 0.5) ;; => 1.2029317631660499
(r/icdf mixture-of-three 0.99) ;; => 10.808075578087667
(r/icdf mixture-of-three ;; => 1.937933121411406
(r/mean mixture-of-three) ;; => 5.7869481264261236
(r/variance mixture-of-three) ;; => ##-Inf
(r/lower-bound mixture-of-three) ;; => ##Inf (r/upper-bound mixture-of-three)
Truncated
name | parameters |
---|---|
|
|
- Name:
:truncated
- Default parameters
:distr
, distribution to truncate:default-normal
:left
, lower boundary:right
, upper boundary
- wiki
By default boundaries are the same as boundaries from a distributions. This way you can make one side truncation.
Please note: derived mean
or variance
is not calculated. Also, set-seed!
doesn’t affect original distribution.
def truncated-normal (r/distribution :truncated {:distr r/default-normal
(:left -2 :right 2}))
def left-truncated-laplace (r/distribution :truncated {:distr (r/distribution :laplace)
(:left -0.5}))
|
|||
|
;; => -0.131952017562289
(r/sample truncated-normal) ;; => 0.41241656836830154
(r/sample truncated-normal) 2.0000001) ;; => 0.0
(r/pdf truncated-normal 2.0) ;; => 0.0565646741125192
(r/pdf truncated-normal 2.0) ;; => 1.0
(r/cdf truncated-normal 0.0) ;; => 0.5000000000000001
(r/cdf truncated-normal map (partial r/icdf truncated-normal) [1.0E-4 0.5 0.9999]) ;; => (-1.9982352293163053 -1.3914582123358836E-16 1.9982352293163053)
(;; => -2.0
(r/lower-bound truncated-normal) ;; => 2.0 (r/upper-bound truncated-normal)
From data
All below distributions can be constructed from datasets or list of values with probabilities.
name | parameters | continuous? |
---|---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Continuous
Continous distribution build from data is based on KDE (Kernel Density Estimation) and PDF integration for CDF and iCDF. Mean and variance are calculated from samples.
:continous-distribution
and :kde
are two names for the same distribution
- Name:
:continuous-distribution
or:kde
- Default parameters:
:data
, samples, sequence of numbers:kde
, density estimation kernel::epenechnikov
:bandwidth
, KDE bandwidth, smoothing parameter:nil
(auto):steps
, number of steps for PDF integration: 5000:min-iterations
, number of PDF integrator iterations:nil
(default):interpolator
, CDF/iCDF interpolator for PDF integration:nil
(default,:linear
)
- wiki, pdf integration
def random-data (repeatedly 1000 (fn [] (+ (* (r/drand -2 2) (r/drand -2 2))
(* (r/drand) (r/drand))))))) (m/sqrt (
def kde-distr (r/distribution :continuous-distribution {:data random-data})) (
|
|||
|
|||
|
|||
|
;; => -0.12303128780717573
(r/sample kde-distr) ;; => -1.6953982126183949
(r/sample kde-distr) 0.0) ;; => 0.2895732291670891
(r/pdf kde-distr 0.0) ;; => 0.31552593767229803
(r/cdf kde-distr map (partial r/icdf kde-distr) [1.0E-4 0.5 0.9999]) ;; => (-4.036565369328684 0.49883262884502827 5.012273231015241)
(;; => -4.1635203556799745
(r/lower-bound kde-distr) ;; => 5.139228217366505
(r/upper-bound kde-distr) ;; => 0.5262235226230889
(r/mean kde-distr) ;; => 1.8233876483481286 (r/variance kde-distr)
Kernels
Distributions for various kernels:
:data
:[-2 -2 -2 -1 0 1 2 -1 0 1 2 0 1 2 1 2 2]
:steps
:100
:bandwidth
: auto
fastmath.kernel/kernel-list
contains three types of kernels: RBF, KDE and what we can call “vector kernels” which includes Marcer, positive definite, and similar.
KDE kernels
Here’s the list of KDE kernels
:cauchy
, :cosine
, :epanechnikov
, :gaussian
, :laplace
, :logistic
, :quartic
, :sigmoid
, :silverman
, :triangular
, :tricube
, :triweight
, :uniform
, :wigner
KDE kernels distribution plots
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
|||
|
Empirical
Empirical distribution calculates PDF, CDF and iCDF from a histogram.
- Name:
:empirical
- Default parameters
:data
, samples, sequence of numbers:bin-count
, number of bins for histogram: 10% of the size of the data
- wiki, source
def empirical-distr (r/distribution :empirical {:data random-data})) (
|
|||
|
;; => 0.8669428900917359
(r/sample empirical-distr) ;; => 1.831863058899185
(r/sample empirical-distr) 0.0) ;; => 0.3367652060230363
(r/pdf empirical-distr 0.0) ;; => 0.3060844150814384
(r/cdf empirical-distr map (partial r/icdf empirical-distr) [1.0E-4 0.5 0.9999]) ;; => (-3.8445695885397138 0.49402601627672765 4.820277450226245)
(;; => -3.8445695885397138
(r/lower-bound empirical-distr) ;; => 4.820277450226245
(r/upper-bound empirical-distr) ;; => 0.5262235226230891
(r/mean empirical-distr) ;; => 1.8233876483481297 (r/variance empirical-distr)
Discrete
- Default parameters
:data
, sequence of numbers (integers/longs or doubles):probabilities
, optional, probabilities or weights
- wiki, source1, source2
Please note: data can contain duplicates.
There are four discrete distributions:
:enumerated-int
for integers, backed by Apache Commons Math:enumerated-real
for doubles, backed by Apache Commons Math:integer-discrete-distribution
- for longs, custom implementation:real-discrete-distribution
- for doubles, custom implementation
Please note:
- Apache Commons Math implementation have some issues with iCDF.
:integer-discrete-distribution
is backed byclojure.data.int-map
Doubles
def data-doubles (repeatedly 100 #(m/sq (m/approx (r/drand 2.0) 1)))) (
|
|||
|
def real-distr (r/distribution :real-discrete-distribution {:data [0.1 0.2 0.3 0.4 0.3 0.2 0.1]
(:probabilities [5 4 3 2 1 5 4]}))
;; => 0.1
(r/sample real-distr) ;; => 0.3
(r/sample real-distr) 0.1) ;; => 0.375
(r/pdf real-distr 0.15) ;; => 0.0
(r/pdf real-distr 0.2) ;; => 0.75
(r/cdf real-distr map (partial r/icdf real-distr) [1.0E-4 0.5 0.9999]) ;; => (0.1 0.2 0.4)
(;; => 0.1
(r/lower-bound real-distr) ;; => 0.4
(r/upper-bound real-distr) ;; => 0.19583333333333333
(r/mean real-distr) ;; => 0.008732638888888894 (r/variance real-distr)
Integers / Longs
def data-ints (repeatedly 500 #(int (m/sqrt (r/drand 100.0))))) (
|
|||
|
def int-distr (r/distribution :integer-discrete-distribution {:data [10 20 30 40 30 20 10]
(:probabilities [5 4 3 2 1 5 4]}))
;; => 10
(r/sample int-distr) ;; => 20
(r/sample int-distr) 10) ;; => 0.375
(r/pdf int-distr 15) ;; => 0.0
(r/pdf int-distr 20) ;; => 0.75
(r/cdf int-distr map (partial r/icdf int-distr) [1.0E-4 0.5 0.9999]) ;; => (10 20 40)
(;; => 10.0
(r/lower-bound int-distr) ;; => 40.0
(r/upper-bound int-distr) ;; => 19.583333333333332
(r/mean int-distr) ;; => 87.32638888888891 (r/variance int-distr)
Categorical
Categorical distribution is a discrete distribution which accepts any data.
- Name:
:categorical-distribution
- Default parameters:
:data
, sequence of any values:probabilities
, optional, probabilities or weights
Order for CDF/iCDF is created by calling (distinct data)
. If sorted data is needed, external sort is necessary. lower-bound
and upper-bound
are not defined though.
def cat-distr (r/distribution :categorical-distribution {:data (repeatedly 100 #(rand-nth [:a :b nil "s"]))})) (
;; => :a
(r/sample cat-distr) ;; => :a
(r/sample cat-distr) nil) ;; => 0.26000000000000006
(r/pdf cat-distr "ss") ;; => 0.0
(r/pdf cat-distr :b) ;; => 0.7300000000000002
(r/cdf cat-distr map (partial r/icdf cat-distr) [1.0E-4 0.5 0.9999]) ;; => (:a nil "s") (
Sequences
Related functions:
sequence-generator
,jittered-sequence-generator
Sequence generators can create random or quasi random vector with certain property like low discrepancy or from ball/sphere.
There are two multimethods:
sequence-generator
, returns lazy sequence of generated vectors (for dim>1) or primitives (for dim=1)jittered-sequence-generator
, returns lazy sequence like above and also add jittering, works only for low discrepancy sequences.
Parameters:
seq-generator
- generator namedimensions
- vector dimensionality, 1 for primitivejitter
- only for jittered sequences, from 0.0 to 1.0, default 0.25
For given dimensionality, returns sequence of:
- 1 - doubles
- 2 -
Vec2
type - 3 -
Vec3
type - 4 -
Vec4
type - n>4 - Clojure vector
Vec2
, Vec3
and Vec4
are fixed size vectors optimized for speed. They act exactly like 2,3 and 4 elements Clojure vectors
Low discrepancy
There are 3 types of sequences:
:sobol
- up to 1000 dimensions, wiki, source:halton
- up to 40 dimensions, wiki, source:r2
- up to 15 dimensions, info
1000 samples from each of the sequence type without and with jittering
:sobol | :halton | :r2 |
![]() |
![]() |
![]() |
:sobol (jittered) | :halton (jittered) | :r2 (jittered) |
![]() |
![]() |
![]() |
first (r/sequence-generator :sobol 4)) ;; => #vec4 [0.0, 0.0, 0.0, 0.0]
(first (r/jittered-sequence-generator :sobol 4)) ;; => #vec4 [0.02900236218752402, 0.03321895859029258, 0.03343024406202439, 0.01735820373097604]
(first (r/sequence-generator :halton 3)) ;; => #vec3 [0.0, 0.0, 0.0]
(first (r/jittered-sequence-generator :halton 3)) ;; => #vec3 [0.00988332128494876, 0.163602670427742, 0.09647432740056015]
(first (r/sequence-generator :r2 2)) ;; => #vec2 [0.2548776662466927, 0.06984029099805333]
(first (r/jittered-sequence-generator :r2 2)) ;; => #vec2 [0.29704197409033695, 0.07653356059721561] (
15 dimensional sequence
take 2 (r/sequence-generator :r2 15)) (
0.45625055763798894
([0.4144151289829652
0.37440997700257395
0.33615502811293263
0.2995737119048001
0.26459280788164197
0.231142298902816
0.19915523103853916
0.16856757955612012
0.13931812076922045
0.11134830949363828
0.08460216186433356
0.05902614327914302
0.03456906124489478
0.01118196291144713]
0.4125011152759779
[0.32883025796593035
0.2488199540051479
0.17231005622586526
0.09914742380960018
0.029185615763283934
0.962284597805632
0.8983104620770783
0.8371351591122402
0.7786362415384409
0.7226966189872766
0.6692043237286671
0.6180522865582859
0.5691381224897896
0.5223639258228941])
One dimensional sequence is just a sequence of numbers
take 20 (r/sequence-generator :sobol 1)) (
0.0
(0.5
0.75
0.25
0.375
0.875
0.625
0.125
0.1875
0.6875
0.9375
0.4375
0.3125
0.8125
0.5625
0.0625
0.09375
0.59375
0.84375
0.34375)
Sphere and ball
Unit sphere or unit ball sequences can generate any dimension.
500 samples
|
|
![]() |
![]() |
first (r/sequence-generator :sphere 4)) ;; => #vec4 [0.5424071626927469, -0.013215716352763234, 0.5218247299811954, -0.6582695237370022]
(first (r/sequence-generator :ball 3)) ;; => #vec3 [0.6106439662537149, -0.19719273807051046, -0.5338472481721354] (
20 dimensional sequence
take 2 (r/sequence-generator :sphere 20)) (
0.10024683329984485
([0.20569256502013525
-0.1555156469751135
-0.08849454905252081
-0.05193412141920333
0.21233505262887162
-0.028799574279578778
0.3737695356904468
0.42139770901601514
-0.02023096283083862
-0.28783014581865857
-0.13014382596383356
-0.07330739388864924
0.06307011647698783
-0.5357026929766503
-0.22318517473179134
-0.13743212701688884
0.04478660464216732
0.058137418049075795
-0.28129202151665705]
0.15809154310731693
[-0.06681106666069823
-0.03215728545808086
0.2857466810468637
0.10136661773650886
-0.026818530655654954
-0.0857147667444245
0.36143042325270464
-0.10584619322452117
-0.44804400894277396
0.3384606363076276
-0.14850501620955514
-0.5199775265327915
0.16910060380195113
-0.060739675348048916
-0.031579757371335546
0.14706770833941443
-0.01162580441478035
-0.05066166738739591
0.24967897944279724]) -
Uniform and Gaussian
Additionally uniform and gaussian N(0,1) sequences can generate any number of dimensions. They rely on default-rng
:default
- uniform distribution U(0,1):gaussian
- gaussian, normal distribution, N(0,1) for each dimension
1000 samples
|
|
![]() |
![]() |
first (r/sequence-generator :default 4)) ;; => #vec4 [0.47512411831706514, 0.9192840007208322, 0.8915903760933624, 0.5999298799277241]
(first (r/sequence-generator :gaussian 3)) ;; => #vec3 [-0.5036463956341344, -0.32895615013785706, 0.4149779826362254] (
Noise
Value, gradient and simplex noises plus various combinations. 1d ,2d and 3d versions are prepared.
Related functions:
single-noise
,fbm-noise
,billow-noise
,ridgemulti-noise
vnoise
,noise
,simplex
random-noise-cfg
,random-noise-fn
discrete-noise
Generation
There are four main methods of noise creation:
single-noise
, single frequency (octave) noisefbm-noise
, multi frequency (octaves), fractal brownian motion *.billow-noise
, multi frequency, “billowy” noiseridgemulti-noise
, multi frequency, ridged multi-fractal
Each noise can be configured in, here is the list of options:
:seed
- seed for noise randomness:noise-type
:value
- value noise:gradient
(default) - gradient noise (Perlin):simplex
- OpenSimplex noise
:interpolation
- interpolation between knots, only for value and gradient noise:none
:linear
:hermite
(default):quintic
:octaves
(default: 6) - number of frequencies/octaves for multi frequency creators:lacunarity
(default: 2) - noise length (1/frequency) for each octave:gain
(default: 0.5) - amplitude factor for each octave:normalize?
(default: true) - if true, range is[0,1]
,[-1,1]
otherwise.
more info about octaves, gain and lacunarity.
Single
def single-g-noise (r/single-noise {:noise-type :gradient :seed 1})) (
0.2) ;; => 0.23759999999999998
(single-g-noise 0.2 0.3) ;; => 0.48526400000000003
(single-g-noise 0.2 0.3 0.4) ;; => 0.660938496 (single-g-noise
Single octave of simplex noise:
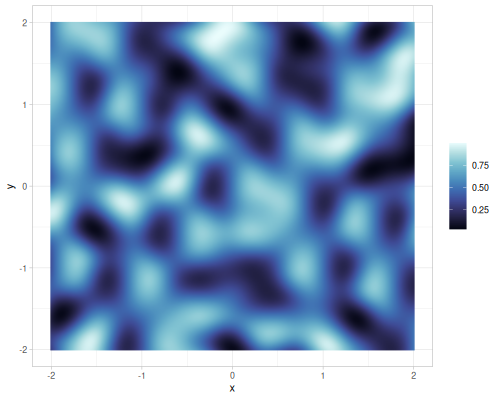
Value and gradient single noise for different interpolations
|
|
|
|
|
value | ![]() |
![]() |
![]() |
![]() |
gradient | ![]() |
![]() |
![]() |
![]() |
FBM
def fbm-noise (r/fbm-noise {:noise-type :gradient :octaves 3 :seed 1})) (
0.2) ;; => 0.7029714285714286
(fbm-noise 0.2 0.3) ;; => 0.44446811428571426
(fbm-noise 0.2 0.3 0.4) ;; => 0.54825344 (fbm-noise
6 octave of simplex noise:
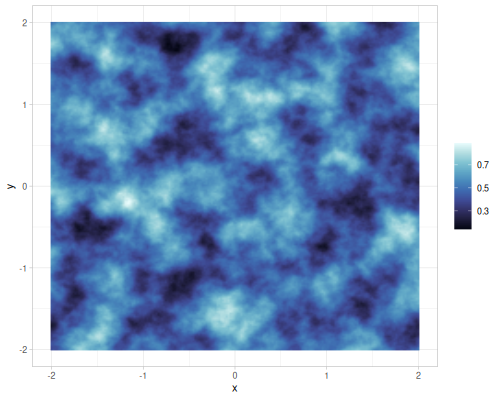
Value and gradient FBM noise for different interpolations
|
|
|
|
|
value | ![]() |
![]() |
![]() |
![]() |
gradient | ![]() |
![]() |
![]() |
![]() |
Different number of octaves for FBM gradient noise
octaves=2 | octaves=4 | octaves=6 | octaves=8 |
![]() |
![]() |
![]() |
![]() |
Different gains and lacunarities for FBM gradient noise
lacunarity=0.5 | lacunarity=2 | lacunarity=5 | lacunarity=8 | |
gain=0.25 | ![]() |
![]() |
![]() |
![]() |
gain=0.5 | ![]() |
![]() |
![]() |
![]() |
gain=0.75 | ![]() |
![]() |
![]() |
![]() |
Billow
def billow-noise (r/billow-noise {:seed 1})) (
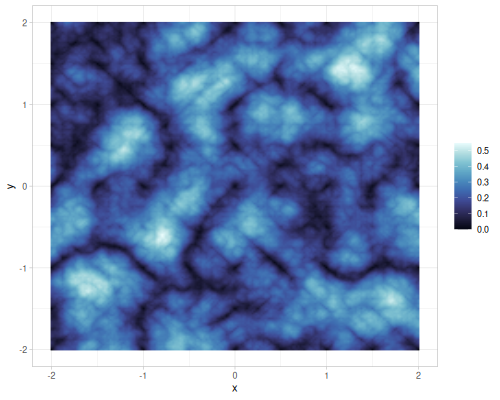
simplex noise | value noise | gradient noise, 1 octave |
![]() |
![]() |
![]() |
0.2) ;; => 0.3879619047619048
(billow-noise 0.2 0.3) ;; => 0.1804361142857142
(billow-noise 0.2 0.3 0.4) ;; => 0.11801290199365083 (billow-noise
Ridged Multi
def ridgedmulti-noise (r/ridgedmulti-noise {:seed 1})) (
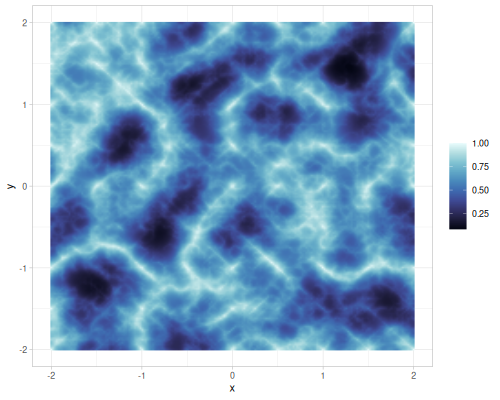
simplex noise | value noise | gradient noise, 1 octave |
![]() |
![]() |
![]() |
0.2) ;; => 0.33387061650044203
(ridgedmulti-noise 0.2 0.3) ;; => 0.6479155481384621
(ridgedmulti-noise 0.2 0.3 0.4) ;; => 0.786227445531883 (ridgedmulti-noise
Predefined
There are three ready to use preconfigured noises:
vnoise
, FBM value noise, 6 octaves, hermite interpolationnoise
, Perlin noise, FBM gradient noise, 6 octaves, quintic interpolationsimplex
, FBM simplex noise, 6 octaves
|
|
|
![]() |
![]() |
![]() |
0.2) ;; => 0.8680365040559659
(r/vnoise 0.2 0.3) ;; => 0.39391987601041634
(r/vnoise 0.2 0.3 0.4) ;; => 0.4321409936990729
(r/vnoise 0.2) ;; => 0.5958232380952381
(r/noise 0.2 0.3) ;; => 0.5299592070095238
(r/noise 0.2 0.3 0.4) ;; => 0.5091397603804436
(r/noise 0.2) ;; => 0.46860690115047615
(r/simplex 0.2 0.3) ;; => 0.4109928995112519
(r/simplex 0.2 0.3 0.4) ;; => 0.5386107648677247 (r/simplex
Warping
Warp noise info
warp-noise-fn
, create warp noise.
Default parameters:
Parameters:
noise
function, default:vnoise
scale
factor, default: 4.0depth
(1 or 2), default 1
|
|
|
|
scale=2 | ![]() |
![]() |
![]() |
scale=4 | ![]() |
![]() |
![]() |
Random configuration
For generative art purposes it’s good to generate random configuration and noise based on it.
random-noise-cfg
, create random configurationrandom-noise-fn
, create random noise from random configuration
Optional parameter is a map with values user wants to fix.
(r/random-noise-cfg)
:interpolation :hermite,
{:warp-scale 0.0,
:seed 665844940,
:normalize? true,
:noise-type :gradient,
:lacunarity 1.90872786462909,
:gain 0.24908653479282347,
:generator :fbm,
:warp-depth 1,
:octaves 6}
:seed 1}) (r/random-noise-cfg {
:interpolation :hermite,
{:warp-scale 4.0,
:seed 1,
:normalize? true,
:noise-type :value,
:lacunarity 1.8874943472437105,
:gain 0.508204375355278,
:generator :fbm,
:warp-depth 1,
:octaves 3}
def some-random-noise (r/random-noise-fn {:seed 1})) (
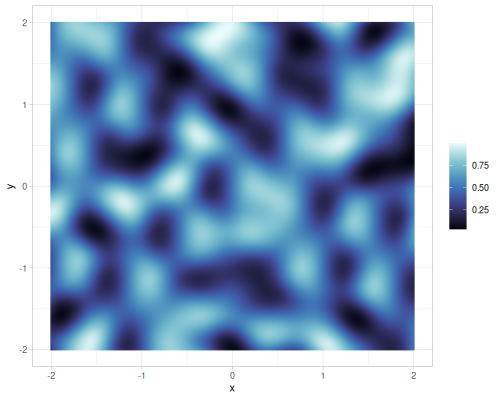
0.2) ;; => 0.32429267456
(some-random-noise 0.2 0.3) ;; => 0.5494103911850483
(some-random-noise 0.2 0.3 0.4) ;; => 0.7304322506666667 (some-random-noise
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
Discrete noise
discrete-noise
is a 1d or 2d hashing function which hashes long or two longs and converts it to a double from [0,1]
range.
100) ;; => 0.07493987729537295
(r/discrete-noise 101) ;; => 0.9625321542669703
(r/discrete-noise 200 100) ;; => 0.6713155553076955
(r/discrete-noise 200 101) ;; => 0.22706653793671472 (r/discrete-noise
Reference
fastmath.random
Various random and noise functions.
Namespace defines various random number generators (RNGs), different types of random functions, sequence generators and noise functions.
### RNGs
You can use a selection of various RNGs defined in Apache Commons Math library.
Currently supported RNGs:
:jdk
- default java.util.Random:mersenne
- MersenneTwister:isaac
- ISAAC:well512a
,:well1024a
,:well19937a
,:well19937c
,:well44497a
,:well44497b
- several WELL variants
To create your RNG use rng multimethod. Pass RNG name and (optional) seed. Returned RNG is equipped with RNGProto protocol with methods: irandom, lrandom, frandom drandom, grandom, brandom which return random primitive value with given RNG.
(let [rng (rng :isaac 1337)]
(irandom rng))
For conveniency default RNG (:jdk
) with following functions are created: irand, lrand, frand, drand, grand, brand.
Each prefix denotes returned type:
- i - int
- l - long
- f - float
- d - double
- g - gaussian (double)
- b - boolean
Check individual function for parameters description.
### Random Vector Sequences
Couple of functions to generate sequences of numbers or vectors.
To create generator call sequence-generator with generator name and vector size. Following generators are available:
:halton
- Halton low-discrepancy sequence; range [0,1]:sobol
- Sobol low-discrepancy sequence; range [0,1]:r2
- R2 low-discrepancy sequence; range [0,1], more…:sphere
- uniformly random distributed on unit sphere:ball
- uniformly random distributed from unit ball:gaussian
- gaussian distributed (mean=0, stddev=1):default
- uniformly random; range:[0,1]
:halton
, :sobol
and :r2
can be also randomly jittered according to this article. Call jittered-sequence-generator.
After creation you get lazy sequence
### Noise
List of continuous noise functions (1d, 2d and 3d):
:value
- value noise:gradient
- gradient noise (improved Ken Perlin version):simplex
- simplex noise
First two (:value
and :gradient
) can use 4 different interpolation types: :none
, :linear
, :hermite
(cubic) and :quintic
.
All can be combined in following variants:
- Noise - pure noise value, create with single-noise
- FBM - fractal brownian motion, create with fbm-noise
- Billow - billow noise, billow-noise
- RidgedMulti - ridged multi, ridgedmulti-noise
Noise creation requires detailed configuration which is simple map of following keys:
:seed
- seed as integer:noise-type
- type of noise::value
,:gradient
(default),:simplex
:interpolation
- type of interpolation (for value and gradient)::none
,:linear
,:hermite
(default) or:quintic
:octaves
- number of octaves for combined noise (like FBM), default: 6:lacunarity
- scaling factor for combined noise, default: 2.00:gain
- amplitude scaling factor for combined noise, default: 0.5:normalize?
- should be normalized to[0,1]
range (true, default) or to[-1,1]
range (false)
For usage convenience 3 ready to use functions are prepared. Returning value from [0,1]
range:
- noise - Perlin Noise (gradient noise, 6 octaves, quintic interpolation)
- vnoise - Value Noise (as in Processing, 6 octaves, hermite interpolation)
- simplex - Simplex Noise (6 octaves)
For random noise generation you can use random-noise-cfg and random-noise-fn. Both can be feed with configuration. Additional configuration:
:generator
can be set to one of the noise variants, defaults to:fbm
:warp-scale
- 0.0 - do not warp, >0.0 warp:warp-depth
- depth for warp (default 1.0, if warp-scale is positive)
#### Discrete Noise
discrete-noise is a 1d or 2d hash function for given integers. Returns double from [0,1]
range.
### Distribution
Various real and integer distributions. See DistributionProto and RNGProto for functions.
To create distribution call distribution multimethod with name as a keyword and map as parameters.
->seq
(->seq)
(->seq rng)
(->seq rng n)
(->seq rng n sampling-method)
Returns lazy sequence of random samples (can be limited to optional n
values).
Additionally one of the sampling methods can be provided, ie: :uniform
, :antithetic
, :systematic
and :stratified
.
ball-random
(ball-random dims)
(ball-random rng dims)
Return random vector from a ball
billow-noise
(billow-noise)
(billow-noise cfg__61329__auto__)
Create billow-noise function with optional configuration.
brand
Random boolean with default RNG.
Returns true or false with equal probability. You can set p
probability for true
brandom
(brandom rng)
(brandom rng p)
Random boolean with provided RNG
ccdf
(ccdf d v)
Complementary cumulative probability.
cdf
(cdf d v)
(cdf d v1 v2)
Cumulative probability.
continuous?
(continuous? d)
Does distribution support continuous domain?
covariance
(covariance d)
Distribution covariance matrix (for multivariate distributions)
default-normal
Default normal distribution (u=0.0, sigma=1.0).
default-rng
Default RNG - JDK
dimensions
(dimensions d)
Distribution dimensionality
discrete-noise
(discrete-noise X Y)
(discrete-noise X)
Discrete noise. Parameters:
- X (long)
- Y (long, optional)
Returns double value from [0,1] range
distribution
Create distribution object.
- First parameter is distribution as a
:key
. - Second parameter is a map with configuration.
All distributions accept rng
under :rng
key (default: default-rng) and some of them accept inverse-cumm-accuracy
(default set to 1e-9
).
distribution-id
(distribution-id d)
Distribution identifier as keyword.
distribution-parameters
(distribution-parameters d)
(distribution-parameters d all?)
Distribution highest supported value.
When all?
is true, technical parameters are included, ie: :rng
and :inverser-cumm-accuracy
.
distribution?
(distribution? distr)
Checks if distr
is a distribution object.
distributions-list
List of distributions.
drand
(drand)
(drand mx)
(drand mn mx)
Random double number with default RNG.
As default returns random double from [0,1)
range. When mx
is passed, range is set to [0, mx)
. When mn
is passed, range is set to [mn, mx)
.
drandom
(drandom rng)
(drandom rng mx)
(drandom rng mn mx)
Random double number with provided RNG
fbm-noise
(fbm-noise)
(fbm-noise cfg__61329__auto__)
Create fbm-noise function with optional configuration.
flip
(flip p)
(flip)
Returns 1 with given probability, 0 otherwise
flip-rng
(flip-rng rng p)
(flip-rng rng)
Returns 1 with given probability, 0 otherwise, for given rng
flipb
(flipb p)
(flipb)
Returns true with given probability, false otherwise
flipb-rng
(flipb-rng rng p)
(flipb-rng rng)
Returns true with given probability, false otherwise, for given rng
frand
(frand)
(frand mx)
(frand mn mx)
Random double number with default RNG.
As default returns random float from [0,1)
range. When mx
is passed, range is set to [0, mx)
. When mn
is passed, range is set to [mn, mx)
.
frandom
(frandom rng)
(frandom rng mx)
(frandom rng mn mx)
Random double number with provided RNG
grand
(grand)
(grand stddev)
(grand mean stddev)
Random gaussian double number with default RNG.
As default returns random double from N(0,1)
. When std
is passed, N(0,std)
is used. When mean
is passed, distribution is set to N(mean, std)
.
grandom
(grandom rng)
(grandom rng stddev)
(grandom rng mean stddev)
Random gaussian double number with provided RNG
icdf
(icdf d v)
Inverse cumulative probability
integrate-pdf
(integrate-pdf pdf-func mn mx steps)
(integrate-pdf pdf-func {:keys [mn mx steps interpolator], :or {mn 0.0, mx 1.0, steps 1000, interpolator :linear}, :as options})
Integrate PDF function, returns CDF and iCDF
Parameters: * pdf-func
- univariate function * mn
- lower bound for integration, value of pdf-func should be 0.0 at this point * mx
- upper bound for integration * steps
- how much subintervals to integrate (default 1000) * interpolator
- interpolation method between integrated points (default :linear)
Also other integration related parameters are accepted (:gauss-kronrod
integration is used).
Possible interpolation methods: :linear
(default), :spline
, :monotone
or any function from fastmath.interpolation
irand
(irand)
(irand mx)
(irand mn mx)
Random integer number with default RNG.
As default returns random integer from full integer range. When mx
is passed, range is set to [0, mx)
. When mn
is passed, range is set to [mn, mx)
.
irandom
(irandom rng)
(irandom rng mx)
(irandom rng mn mx)
Random integer number with provided RNG
jittered-sequence-generator
(jittered-sequence-generator seq-generator dimensions)
(jittered-sequence-generator seq-generator dimensions jitter)
Create jittered sequence generator.
Suitable for :r2
, :sobol
and :halton
sequences.
jitter
parameter range is from 0
(no jitter) to 1
(full jitter). Default: 0.25.
See also sequence-generator.
likelihood
(likelihood d vs)
Likelihood of samples
log-likelihood
(log-likelihood d vs)
Log likelihood of samples
lower-bound
(lower-bound d)
Distribution lowest supported value
lpdf
(lpdf d v)
Log density
lrand
(lrand)
(lrand mx)
(lrand mn mx)
Random long number with default RNG.
As default returns random long from full integer range. When mx
is passed, range is set to [0, mx)
. When mn
is passed, range is set to [mn, mx)
.
lrandom
(lrandom rng)
(lrandom rng mx)
(lrandom rng mn mx)
Random long number with provided RNG
mean
(mean d)
Distribution mean
means
(means d)
Distribution means (for multivariate distributions)
noise
(noise x)
(noise x y)
(noise x y z)
Improved Perlin Noise.
6 octaves, quintic interpolation.
noise-generators
List of possible noise generators as a map of names and functions.
noise-interpolations
List of possible noise interpolations as a map of names and values.
noise-types
List of possible noise types as a map of names and values.
observe MACRO
(observe d vs)
Log likelihood of samples. Alias for log-likelihood.
observe1
(observe1 d v)
Log of probability/density of the value. Alias for lpdf.
(pdf d v)
Density
probability
(probability d v)
Probability (PMF)
random-noise-cfg
(random-noise-cfg pre-config)
(random-noise-cfg)
Create random noise configuration.
Optional map with fixed values.
random-noise-fn
(random-noise-fn cfg)
(random-noise-fn)
Create random noise function from all possible options.
Optionally provide own configuration cfg
. In this case one of 4 different blending methods will be selected.
randval MACRO
(randval v1 v2)
(randval prob v1 v2)
(randval prob)
(randval)
Return value with given probability (default 0.5)
randval-rng MACRO
(randval-rng rng v1 v2)
(randval-rng rng prob v1 v2)
(randval-rng rng prob)
(randval-rng rng)
Return value with given probability (default 0.5), for given rng
ridgedmulti-noise
(ridgedmulti-noise)
(ridgedmulti-noise cfg__61329__auto__)
Create ridgedmulti-noise function with optional configuration.
rng
Create RNG for given name (as keyword) and optional seed. Return object enhanced with RNGProto. See: rngs-list for names.
rngs-list
List of all possible RNGs.
roll-a-dice
(roll-a-dice sides)
(roll-a-dice dices sides)
Roll a dice with given sides
roll-a-dice-rng
(roll-a-dice-rng rng sides)
(roll-a-dice-rng rng dices sides)
Roll a dice with given sides and given rng
sample
(sample d)
Random sample
sequence-generator
Create Sequence generator. See sequence-generators-list for names.
Values:
:r2
,:halton
,:sobol
,:default
/:uniform
- range[0-1] for each dimension
:gaussian
- fromN(0,1)
distribution:sphere
- from surface of unit sphere (ie. euclidean distance from origin equals 1.0):ball
- from an unit ball
Possible dimensions:
:r2
- 1-15:halton
- 1-40:sobol
- 1-1000- the rest - 1+
See also jittered-sequence-generator.
sequence-generators-list
List of random sequence generator. See sequence-generator.
set-seed
(set-seed)
(set-seed v)
(set-seed rng v)
Create and return new RNG
set-seed!
(set-seed!)
(set-seed! v)
(set-seed! rng v)
Sets seed.
simplex
(simplex x)
(simplex x y)
(simplex x y z)
Simplex noise. 6 octaves.
single-noise
(single-noise)
(single-noise cfg__61329__auto__)
Create single-noise function with optional configuration.
source-object
(source-object d)
Returns Java or proxy object from backend library (if available)
synced-rng
(synced-rng m)
(synced-rng m seed)
Create synchronized RNG for given name and optional seed. Wraps rng method.
upper-bound
(upper-bound d)
Distribution highest supported value
variance
(variance d)
Distribution variance
vnoise
(vnoise x)
(vnoise x y)
(vnoise x y z)
Value Noise.
6 octaves, Hermite interpolation (cubic, h01).
warp-noise-fn
(warp-noise-fn noise scale depth)
(warp-noise-fn noise scale)
(warp-noise-fn noise)
(warp-noise-fn)
Create warp noise (see Inigo Quilez article).
Parameters:
- noise function, default: vnoise
- scale factor, default: 4.0
- depth (1 or 2), default 1
Normalization of warp noise depends on normalization of noise function.
source: clay/random.clj