fastmath.complex
Complex numbers functions.
Complex number is represented as Vec2
type (from clojure2d.math.vector namespace).
To create complex number use complex, vec2 or ->Vec2.
Implementation checks for ##Inf, ##NaN and some of the function distinguish +0.0 and -0.0
Categories
Other vars: -I I I- ONE TWO ZERO abs acos acosh acot acoth acsc acsch add arg asec asech asin asinh atan atanh complex conjugate cos cosh cot coth csc csch csgn delta-eq div exp flip im imaginary? inf? log logb mult mult-I mult-I- nan? neg norm pow re real? reciprocal scale sec sech sin sinh sq sqrt sqrt1z sub tan tanh zero?
abs
(abs z)
Absolute value, magnitude
Examples
Abs
(abs (complex 1 -3))
;;=> 3.1622776601683795
acos
(acos z)
acos
Examples
acos(z)
(acos (complex 2 -1))
;;=> [0.5073563032171444 1.4693517443681854]
Plot of acos
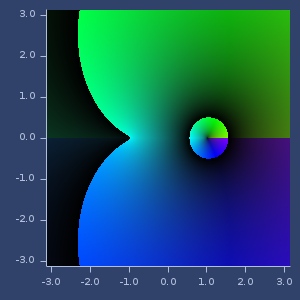
arg
(arg z)
Argument (angle) of the complex number
Examples
Argument
(m/degrees (arg I-))
;;=> -90.0
asin
(asin z)
asin
Examples
asin(z)
(asin (complex 2 -1))
;;=> [1.0634400235777521 -1.4693517443681854]
Plot of asin
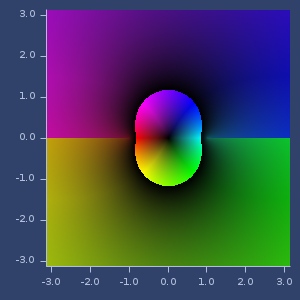
atan
(atan z)
atan
Examples
atan(z)
(atan (complex 2 -1))
;;=> [1.1780972450961724 -0.17328679513998632]
Plot of atan
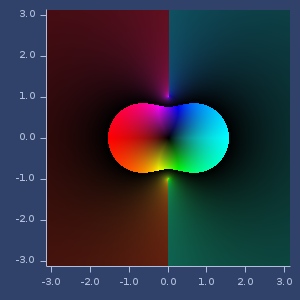
complex
(complex a b)
(complex a)
(complex)
Create complex number. Represented as Vec2
.
Examples
New complex number.
(complex 2 -1)
;;=> [2.0 -1.0]
conjugate
(conjugate z)
Complex conjugate. \(\bar{z}\)
Examples
Conjugate
(conjugate I)
;;=> [0.0 -1.0]
cos
(cos z)
cos
Examples
cos(z)
(cos (complex 2 -1))
;;=> [-0.6421481247155197 1.0686074213827785]
Plot of cos
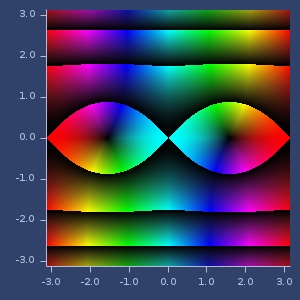
cosh
(cosh z)
cosh
Examples
cosh(z)
(cosh (complex 2 -1))
;;=> [2.0327230070196656 -3.0518977991517997]
Plot of cosh
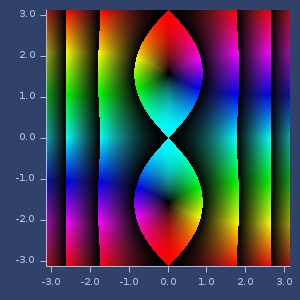
csc
(csc z)
csc
Examples
csc(z)
(csc (complex 2 -1))
;;=> [0.6354937992538998 -0.22150093085050923]
Plot of csc
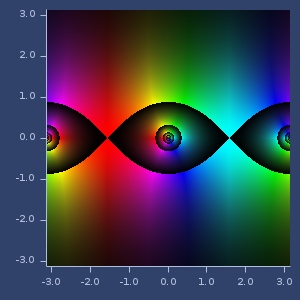
csgn
(csgn re im)
(csgn z)
Complex sgn.
Returns 0
for 0+0i
or calls m/sgn
on real part otherwise.
delta-eq
(delta-eq q1 q2)
(delta-eq q1 q2 accuracy)
Compare complex numbers with given accuracy (10e-6 by default)
div
(div z1 z2)
Divide two complex numbers.
Examples
Divide
(div (complex 1 2) (complex 3 4))
;;=> [0.44 0.08]
exp
(exp z)
exp
Examples
exp(z)
(exp (complex 2 -1))
;;=> [3.992324048441272 -6.217676312367968]
\(e^{i\pi}+1\)
(add (exp (complex 0 m/PI)) ONE)
;;=> [0.0 1.224646799076922E-16]
Plot of exp
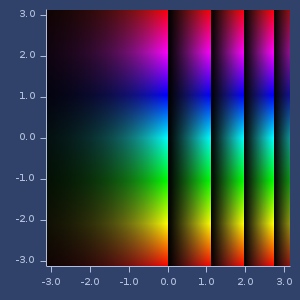
log
(log z)
log, principal value
Examples
log(z)
(log (complex 2 -1))
;;=> [0.8047189562170501 -0.4636476090008061]
log(e)
(log (complex m/E 0))
;;=> [1.0 0.0]
Plot of log
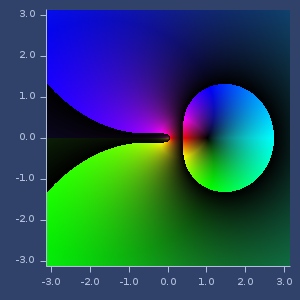
mult
(mult z1 z2)
Multiply two complex numbers.
Examples
Multiply
(mult (complex 1 2) (complex 3 4))
;;=> [-5.0 10.0]
neg
(neg z)
Negate complex number. \(-z\)
Examples
Negate.
(neg (complex 1 2))
;;=> [-1.0 -2.0]
pow
(pow z1 z2)
Power. \(z_1^{z_2}\)
Examples
\(\sqrt{2}\)
(pow TWO (complex 0.5 0.0))
;;=> [1.4142135623730951 0.0]
Complex power
(pow (complex 1 2) (complex 3 4))
;;=> [0.12900959407446694 0.03392409290517002]
reciprocal
(reciprocal z)
\(\frac{1}{z}\)
Examples
Reciprocal of real
(reciprocal TWO)
;;=> [0.5 -0.0]
Reciprocal of complex
(reciprocal (complex 0 2))
;;=> [0.0 -0.5]
Plot of reciprocal
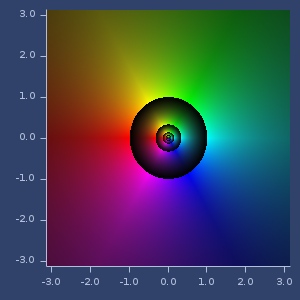
sec
(sec z)
sec
Examples
sec(z)
(sec (complex 2 -1))
;;=> [-0.4131493442669398 -0.687527438655479]
Plot of sec
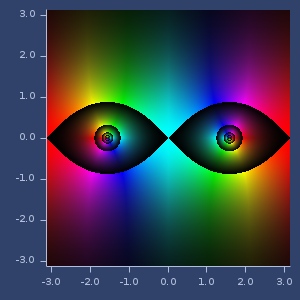
sin
(sin z)
sin
Examples
sin(z)
(sin (complex 2 -1))
;;=> [1.403119250622041 0.4890562590412935]
Plot of sin
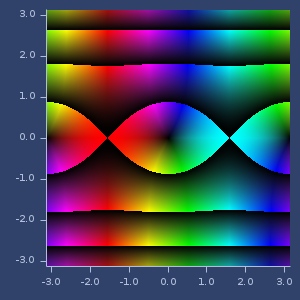
sinh
(sinh z)
sinh
Examples
sinh(z)
(sinh (complex 2 -1))
;;=> [1.9596010414216063 -3.1657785132161678]
Plot of sinh
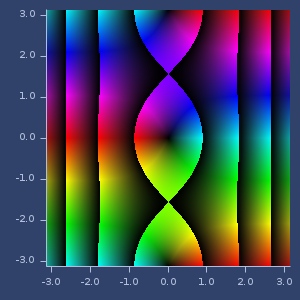
sq
(sq z)
Square complex number. \(z^2\)
Examples
Square.
(sq (complex 1 2))
;;=> [-3.0 4.0]
\(i^2\)
(sq I)
;;=> [-1.0 0.0]
Plot of sq
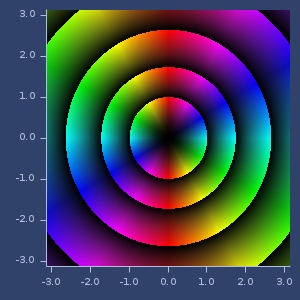
sqrt
(sqrt z)
Sqrt of complex number. \(\sqrt{z}\)
Examples
Square root of real.
(sqrt (complex 2 0))
;;=> [1.4142135623730951 0.0]
Square root of complex.
(sqrt (complex 2 2))
;;=> [1.5537739740300376 0.6435942529055827]
Plot of sqrt
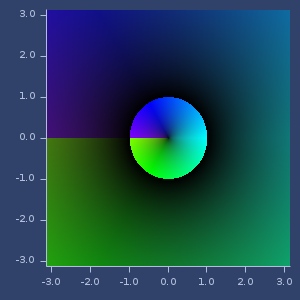
sqrt1z
(sqrt1z z)
\(\sqrt{1-z^2}\)
Examples
Example 1
(sqrt1z (complex 2 3))
;;=> [3.115799084103365 -1.9256697360916721]
Plot of sqrt1z
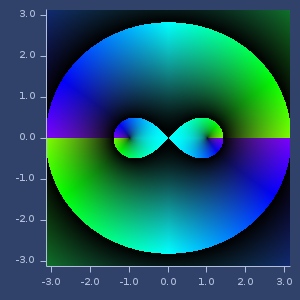
sub
(sub z1 z2)
Difference of two complex numbers
Examples
Subtract
(sub ONE I-)
;;=> [1.0 1.0]
tan
(tan z)
tan
Examples
tan(z)
(tan (complex 2 -1))
;;=> [-0.24345820118572514 -1.16673625724092]
Plot of tan
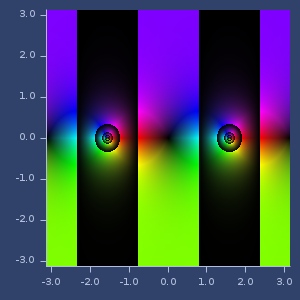
tanh
(tanh z)
tanh
Examples
tanh(z)
(tanh (complex 2 -1))
;;=> [1.0147936161466335 -0.033812826079896684]
Plot of tanh
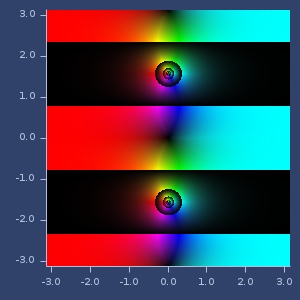